A simple example of servlet coding:
To illustrate the previous discussion, we shall consider how to write a servlet that will respond to a simple request for dynamically generated data. The example we shall consider is a request for a set of randomly generated numbers, such as might be required for a lottery. In a lottery, participants normally have to select a set of numbers with each number being within a given range. They also have to pay a small amount to enter the lottery. A central system then randomly generates a set of winning numbers, and the one or more people who chose exactly those numbers win or share the lottery prize, which is often a large sum of money.
In this first example, the client system will ask the server to respond with a suitable set of numbers to be entered in the lottery. At this stage, we assume that the rest of the lottery process is handled elsewhere - this system simply helps the lottery player to identify a set of suitable 'lucky' numbers. Later we will add more features, making the system a little more realistic.
This lottery requires players to choose a set of four integers, each between 1 and 99 inclusive. Hence, all the servlet needs to do is to generate four suitable random integers and to return these on a web page to the client. Admittedly, we could just as easily generate these numbers using an applet, or in JavaScript, but consider this simple servlet as a base for further functions to be added later. For simplicity, we ignore the (real) possibility of duplicate random numbers being generated.
As we have seen, a servlet is written as a class that extends the abstract HttpServlet class. In this case we expect the client to issue an HTTP GET request, so we must override the doGet method to provide a suitable web page in response.
The code for the LotteryLuckyNumbersServlet is as follows.
public class LotteryLuckyNumbersServlet extends HttpServlet
{
final static int NUMBER_OF_VALUES = 4;
final static int MAX_VALUE = 99; // numbers from 1 to this
Random random; // random number generator
int [] value; // lucky number values
/ * Initialize the random number generator and set up an array for the lucky numbers. */
public void init ( )
{
random = new Random( );
value = new int [NUMBER_OF_VALUES ];
}
public void doGet (HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException
{
// generate the random lucky numbers
for (int i = 0; i < NUMBER_OF_VALUES; i++)
{
value [i ] = random.nextInt(MAX_VALUE) + 1;
}
// send them back in a web page resp.setContentType("text/HTML");
PrintWriter out = resp.getWriter( );
out.println("<HTML>");
out.println("<HEAD>");
out.println("<TITLE>Lucky Numbers</TITLE>");
out.println("</HEAD>"); out.println("<BODY>");
out.println("<H1>Walrus Lottery</H1>");
out.println("<BR> <BR>");
out.println("<H2>Your lucky numbers are:</H2>");
out.println("<BR> <BR> <H1>");
for (int i = 0; i < NUMBER_OF_VALUES; i++)
{
out.print (value [i ] + " ");
}
out.println("</H1>");
out.println("<BR> <BR>");
out.println("<H2>Wishing you the best of luck</H2>");
out.println("<BR> <HR> <BR>");
out.println("<H4>We do not guarantee a winning result</H4>");
out.println("<H4>Walruses can go down as well as up</H4>");
out.println("</BODY>");
out.println("</HTML>");
out.flush( );
out.close( );
}
}
The class defines two public methods, init and doGet, each of which overrides a default implementation. The init method runs once when the servlet is first activated. In this case, it creates and initializes the random number generator object as well as setting up an array to hold the lucky numbers.
The doGet method constructs and sends the response to a GET request from the client. This method has two arguments, req and resp, that reference objects corresponding to the request data received and the response to be constructed, respectively. In this simple case, there is no additional data associated with the request so the req argument is not used. The response object is used to send the reply.
In this case, there is no need to override the destroy method as there are no resources to release apart from the objects created by the init method - these will be dealt with by Java garbage collection in the normal way.
The servlet sends back an HTTP response consisting of a header, indicating the type of content (text and HTML), followed by the source of the web page as follows:
<HTML>
<HEAD>
<TITLE>Lucky Numbers</TITLE>
</HEAD>
<BODY>
<H1>Walrus Lottery</H1>
<BR> <BR>
<H2>Your lucky numbers are:</H2>
<BR> <BR>
<H1>4 21 52 47</H1>
<BR> <BR>
<H2>Wishing you the best of luck</H2>
<BR> <HR> <BR>
<H4>We do not guarantee a winning result</H4>
<H4>Walruses can go down as well as up</H4>
</BODY>
</HTML>
Of course, the lucky numbers that are returned will vary with each response. This is why a static web page cannot be used in this case. When viewed using a browser, this web page is as shown in Figure.
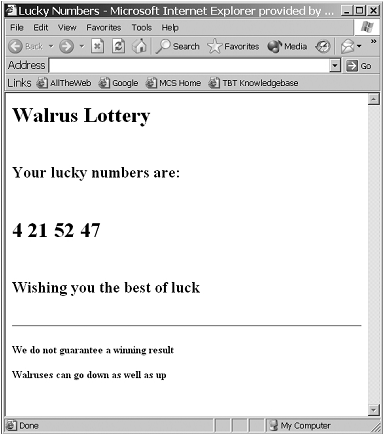
Figure: The lucky numbers web page returned by the
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your A simple example of servlet coding homework and assignments? Live A simple example of servlet coding experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer A simple example of servlet coding homework help, java assignment help and A simple example of servlet coding projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours