A ball game in Java:
The final example pulls together some of the ideas that we have met so far in this unit to create a simple ball game. It involves the user using the left and right arrow keys to move a 'paddle' in order to hit a moving ball. When the player hits the moving ball, then it bounces off and hits the sides of the box until it comes down again. The object of the game is to keep the ball 'up'. There is no scoring mechanism and the user has to run the program again to get another go. However, it includes almost all of the key elements of a game. The operation of the game is a little 'jerky' because the computer is checking for user input and moving the ball at the 'same time'. In Unit 8 we will see a much more sophisticated way of getting the computer to do 'more than one thing at a time'.
The complete code for the various classes is given below. Some of the classes have been changed slightly. We will alert you to these changes and explain them when they occur.
The first class, BallGame, is a subclass of MovingBall, although the version of MovingBall that we are using is slightly different from the one we used before in Subsection. The class BallGame essentially deals with the user interaction using the KeyAdapter and checks to see whether the ball and the paddle, as shown in Figure, are sufficiently close to cause the ball to bounce.
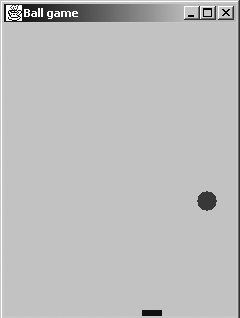
Figure: The BallGame frame showing the ball and paddle
public class BallGameTest
{
public static void main (String [] args)
{
BallGame world = new BallGame("Ball game");
world.setVisible(true);
world.move( );
}
}
import java.awt.Graphics;
import java.awt.event. *;
public class BallGame extends MovingBall
{
private Paddle myPaddle = new Paddle(FRAME_WIDTH, FRAME_HEIGHT);
public BallGame (String title)
{
super(title);
addKeyListener(new KeyList( ));
}
public void paint (Graphics g)
{
super.paint(g);
if (isContact( ))
{
myBall.bounce( );
}
else
{
myPaddle.paint(g);
}
}
public boolean isContact( )
{
if (myPaddle.area( ).contains(myBall.getPosition( )))
{
return true;
}
else
{
return false;
}
}
// inner class for the listener
private class KeyList extends KeyAdapter
{
public void keyPressed (KeyEvent k)
{
if (k.getKeyCode( ) == KeyEvent.VK_LEFT)
{
myPaddle.moveLeft( );
}
if (k.getKeyCode( ) == KeyEvent.VK_RIGHT)
{
myPaddle.moveRight( );
}
} // ends method keyPressed
} // ends private class KeyList
} // ends class BallGame
In this version of MovingBall, some of the private fields have been changed to protected in order to allow subclasses to be created. Furthermore, instead of using CloseAndQuit, we illustrate the use of the setDefaultCloseOperation(JFrame. EXIT_ON_CLOSE) statement.
import java.awt. *; import javax.swing. *; import java.awt.event. *;
public class MovingBall extends JFrame
{
protected final int FRAME_WIDTH = 240;
protected final int FRAME_HEIGHT = 320;
protected Ball myBall = new Ball(FRAME_WIDTH, FRAME_HEIGHT);
public MovingBall (String title)
{
super(title);
setSize(FRAME_WIDTH, FRAME_HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void paint (Graphics g)
{
super.paint(g);
myBall.paint(g);
}
public void move ( )
{
while (true)
{
myBall.move( );
repaint( );
try
{
Thread.sleep(50);
}
catch (InterruptedException e)
{
System.exit(0);
}
} // ends while
} // ends move
} // ends MovingBall
Below is the Ball class. The main changes to Ball are those to the move method and in the introduction of a new method, bounce. These methods cause the ball to 'bounce' off the sides of the frame as well as off the paddle if this is in the right place at the right time. A more sophisticated system could incorporate some 'noise' into the bounce, for example, but here we have implemented a simple version.
import java.awt. *;
public class Ball
{
private final int RADIUS = 10;
private Point pos;
private Color ballColour = Color.red;
private int yChange = 2;
private int xChange = 1;
private int height, width;
public Ball (int frameWidth, int frameHeight)
{
width = frameWidth; height = frameHeight; pos = new Point ( );
pos.x = (int)(Math.random( ) * (width - RADIUS)) + RADIUS;
pos.y = (int)(Math.random( ) * (height/2 - RADIUS)) + RADIUS;
}
public void paint (Graphics g)
{
g.setColor(ballColour);
g.fillOval(pos.x - RADIUS,
pos.y - RADIUS, 2 * RADIUS, 2 * RADIUS);
}
public void move ( )
{
if (pos.y < RADIUS)
{
yChange = -yChange;
}
if (pos.x < RADIUS)
{
xChange = -xChange;
}
if (pos.x > width - RADIUS)
{
xChange = -xChange;
}
if (pos.y < height - RADIUS)
{
pos.translate(xChange, yChange);
}
if (pos.x < width - RADIUS)
{
pos.translate(xChange, yChange);
}
}
public void bounce ( )
{
yChange = -yChange;
pos.translate(xChange, yChange);
}
public Point getPosition ( )
{
return pos;
}
}
The final class is Paddle. This acts as the 'paddle' that the user moves along the bottom of the frame. As well as knowing how to paint itself and how to move to the left or to the right, it has a method called area that helps it to 'know its own extent'. The method area works by creating a Rectangle at the current position of the paddle. This Rectangle object is then used in the class BallGame, which is in the method isContact, to decide whether the ball and the paddle are in the same space and whether the ball should be instructed to bounce.
import java.awt. *;
import java.awt.event. *;
import javax.swing. *;
public class Paddle
{
private Color paddleColour = Color.blue;
private int x, y;
private int paddleWidth = 20;
private int paddleHeight = 10;
private int move = 5;
public Paddle (int frameWidth,int frameHeight)
{
x = (int)(Math.random( ) * (frameWidth - paddleWidth));
y = frameHeight - paddleHeight;
}
public void moveRight ( )
{
x = x + move;
}
public void moveLeft ( )
{
x = x - move;
}
public Rectangle area ( )
{
return new Rectangle(x, y, paddleWidth, paddleHeight);
}
public void paint (Graphics g)
{
g.setColor(paddleColour);
g.fillRect(x, y, paddleWidth, paddleHeight);
}
}
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your A ball game in Java homework and assignments? Live A ball game in Java experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer A ball game in Java homework help, java assignment help and A ball game in Java projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours